Simple Predator/Prey Modeling (먹이사슬 모델링)
포스트 난이도: HOO_Middle
# Example Codes
생물뿐만 아니라 관계성이 있는 모델링을 할 때 주로 고려하는 모델링 방식 중 하나가 바로 Predator/Prey modeling이다. 상관관계가 있다면 활용이 가능하고 경우에 따라서 수를 늘려나갈 수도 있기 때문에 모델링 개발에 있어서 용이하다. 이번 포스트에서는 간단한 먹이사슬 모델링을 파이썬 코드로 살펴보도록 하자.
이번 예제코드는 간단한 예시이기에 Euler와 Lotka-Volterra model를 통해서 표현해 보았다.
import numpy as np
import matplotlib.pyplot as plt
# Constants
r = 0.1 # Prey reproduction rate
g = 0.002 # Predator reproduction rate
m = 0.2 # Predator mortality rate
h = 0.0002 # Prey-predator interaction rate
# Initial values
N0 = 1500 # Initial prey population
P0 = 50 # Initial predator population
# Simulation parameters
dt = 0.1 # Time step
num_steps = 2000 # Number of time steps
# Initialize time array
time = np.arange(0, num_steps * dt, dt)
# Lotka-Volterra model equations
def lv_model(X, t, alpha, beta, delta, gamma):
N, P = X
dNdt = alpha * N - beta * N * P
dPdt = delta * N * P - gamma * P
return np.array([dNdt, dPdt])
# Perform numerical integration using the Euler method
alpha = r
beta = h
delta = g
gamma = m
X0 = [N0, P0]
X = Euler(lv_model, X0, time, alpha, beta, delta, gamma)
# Extract prey and predator populations
prey_population = X[:, 0]
predator_population = X[:, 1]
# Plotting the first graph (Density vs. Time)
plt.figure(figsize=(12, 6))
plt.subplot(121)
plt.plot(time, prey_population, label='Prey Density', color='green')
plt.plot(time, predator_population, label='Predator Density', color='red')
plt.xlabel('Time')
plt.ylabel('Density')
plt.legend()
plt.title('Density of Prey vs Predator')
# Plotting the second graph (Phase Plot)
plt.subplot(122)
plt.plot(prey_population, predator_population, label='Phase Plot', color='blue')
plt.xlabel('Prey Density')
plt.ylabel('Predator Density')
plt.title('Phase Plot of Predator Density vs. Prey Density')
# Plot predator and prey isoclines
prey_isocline = np.linspace(0, max(prey_population), num_steps)
predator_isocline = alpha / beta + np.zeros(num_steps)
plt.plot(prey_isocline, predator_isocline, '--', label='Prey Isocline', color='green')
plt.plot(prey_isocline, predator_isocline * (delta / gamma), '--', label='Predator Isocline', color='red')
plt.legend()
plt.tight_layout()
plt.show()
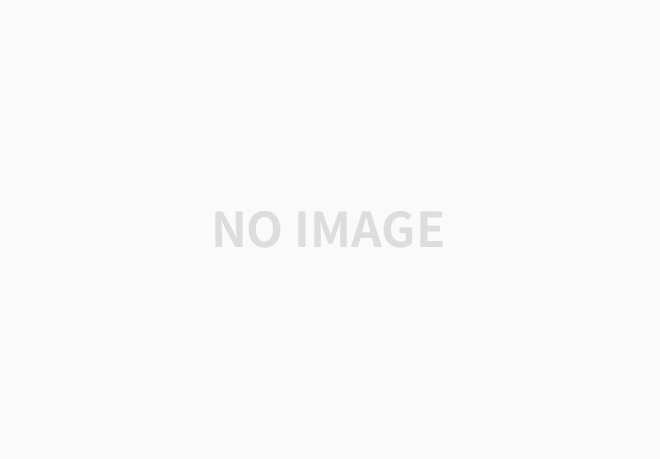
# github link
https://github.com/WhoisHOO/HOOAI/blob/main/Python%20Examples/lotka-Volterra_model
728x90
댓글